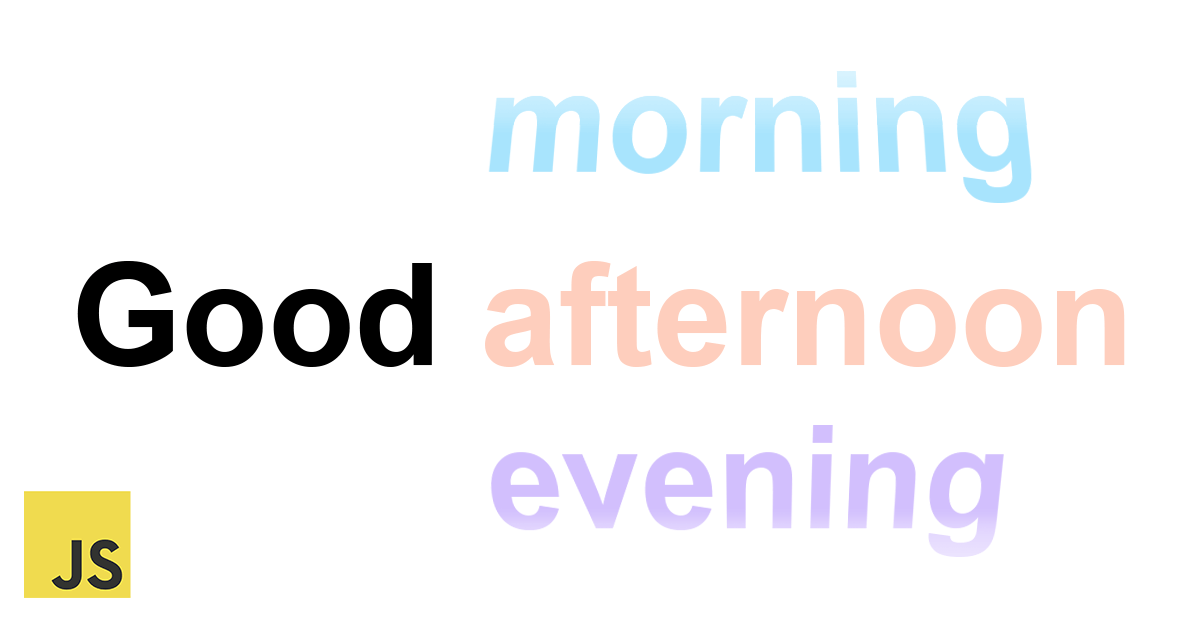
In order to make a local, time-based message (let's say for a greeting) that will display a message to the user based on their current time, you will need new Date();
. Once you have that, the world is your Cloyster!
Let's take a look!
try { // Get the current date from browser var now = new Date(); } catch (err) { console.log("Error: Could not get date. No soup for you!" + err.message ); }
This try-catch block tries to grab the date with new Date();
and save it to the variable now
. If there is an error it will log to the browser's console (not visible on the page, in the browser dev-tools) a saucy message plus the actual error. Cool!
if (now != null) { var hour = now.getHours(); if (hour >= 0 && hour <= 5) { // 12am - 5am document.getElementById("greeting").innerHTML = "Shhh... it's past midnight"; } if (hour >= 6 && hour <= 11) { // 6am - 11am document.getElementById("greeting").innerHTML = "Good morning"; } if (hour >= 12 && hour <= 16) { // 12pm - 4pm document.getElementById("greeting").innerHTML = "Good afternoon"; } if (hour >= 17 && hour <= 23) { // 5pm - 11pm document.getElementById("greeting").innerHTML = "Good evening"; } }
The last section starts with first checking if now
doesn't equal null
, implying that it couldn't get the date. If it has data in the now
variable, it will proceed, if not, it can hit the road. Anyways, if now
has data, we will get the current hour with now.getHours();
and save that to the variable hour
. Next, we just need to define some if
statements that define the slices of hours (greater or equal to this AND less or equal to that). BAM! That's it! Well, sort of... you need to define an HTML element (in this example greeting
) to put the greeting in, sprinkle in some CSS, and you are good to go!
<html> <style> .timegreeting{ font-family: "arial", sans-serif; font-weight: bold; font-size: 50px; } </style> <p id="greeting" class="timegreeting"></p> <script> try { // Get the current date from browser var now = new Date(); } catch (err) { console.log("Error: Could not get date. No soup for you!" + err.message ); } if (now != null) { var hour = now.getHours(); if (hour >= 0 && hour <= 5) { // 12am - 5am document.getElementById("greeting").innerHTML = "Shhh... it's past midnight"; } if (hour >= 6 && hour <= 11) { // 6am - 11am document.getElementById("greeting").innerHTML = "Good morning"; } if (hour >= 12 && hour <= 16) { // 12pm - 4pm document.getElementById("greeting").innerHTML = "Good afternoon"; } if (hour >= 17 && hour <= 23) { // 5pm - 11pm document.getElementById("greeting").innerHTML = "Good evening"; } } </script> </html>